One of the things we commonly need is a list of user info. Putting that together in code is easy – but if we want a nice output to send to users? This does that.
"
$line = "
|
"
$TableRow = "
"
# Cell
$CellStart = "
"
$Cellmiddle2 = " |
"
$cellend = " |
"
$defaultcolorCell = $TableBody + $CellStart + $Goodgreen + $cellMiddle1
### example
# $DistinguishedNameTitle = "Distinguished Name:"
# $DistinguishedNameColor = $Goodgreen
# $DistinguishedNamedata = $DistinguishedName
# $DistinguishedNameCells = $CellStart + $DistinguishedNameColor + $cellMiddle1 + $DistinguishedNameTitle + $Cellmiddle2 + $DistinguishedNamedata + $cellend
# $TableBody = $TableBody + $CellStart + $DistinguishedNameColor + $cellMiddle1 + $DistinguishedNameTitle + $Cellmiddle2 + $DistinguishedNamedata + $cellend
############################
##
## Data to return
##
############################
# start our Table body with a new line and a row
[string]$TableBody = $line + $TableRow
##################################################
# Who info
$name = $ObjUser.DisplayName
$nametitle = "Display Name: "
$namedata = $ObjUser.DisplayName
$TableBody = $TableBody + $defaultcolorCell + $NameTitle + $Cellmiddle2 + $Namedata + $cellend
$UserSAM = $objuser.SamAccountName
$UserSAMtitle = "Login: "
$UserSAMdata = $UserSAM
$TableBody = $TableBody + $defaultcolorCell + $UserSAMtitle + $Cellmiddle2 + $UserSAMdata + $cellend
#new Row
$TableBody = $TableBody + $TableRow
$title = $objuser.Title
$titletitle = "Title: "
$titledata = $title
$TableBody = $TableBody + $defaultcolorCell + $titletitle + $Cellmiddle2 + $titledata + $cellend
$Description = $ObjUser.Description
$Descriptiontitle = "Prefered Title: "
$Descriptiondata = $Description
$TableBody = $TableBody + $defaultcolorCell + $Descriptiontitle + $Cellmiddle2 + $Descriptiondata + $cellend
#new Row
$TableBody = $TableBody + $TableRow
$employeedepartment = $ObjUser.Department
$employeedepartmenttitle = "Department: "
$employeedepartmentdata = $employeedepartment
$TableBody = $TableBody + $defaultcolorCell + $employeedepartmenttitle + $Cellmiddle2 + $employeedepartmentdata + $cellend
$Company = $ObjUser.Company
$Companytitle = "Company: "
$Companydata = $Company
$TableBody = $TableBody + $defaultcolorCell + $Companytitle + $Cellmiddle2 + $Companydata + $cellend
#new Row
$TableBody = $TableBody + $TableRow
$DistinguishedName = $ObjUser.DistinguishedName
$DistinguishedNameTitle = "Distinguished Name: "
$DistinguishedNamedata = $ObjUser.DistinguishedName
$TableBody = $TableBody + $defaultcolorCell + $DistinguishedNameTitle + $Cellmiddle2 + $DistinguishedNamedata + $cellend
##################################################
# Employee Details
#new Line
$TableBody = $TableBody + $line
#Section header
$TableBody = $TableBody + "
EMPLOYEE DETAILS |
"
#new Line
$TableBody = $TableBody + $line
#new Row
$TableBody = $TableBody + $TableRow
$officephone = $objuser.OfficePhone
$officephonetitle = "Office Phone: "
$officephonedata = $officephone
$TableBody = $TableBody + $defaultcolorCell + $officephonetitle + $Cellmiddle2 + $officephonedata + $cellend
$homephone = $ObjUser.HomePhone
$homephonetitle = "Home or Cell: "
$homephonedata = $homephone
$TableBody = $TableBody + $defaultcolorCell + $homephonetitle + $Cellmiddle2 + $homephonedata + $cellend
#new Row
$TableBody = $TableBody + $TableRow
$employeeID = $objuser.EmployeeID
$employeeIDtitle = "Employee ID: "
$employeeIDdata = $employeeID
$TableBody = $TableBody + $defaultcolorCell + $employeeIDtitle + $Cellmiddle2 + $employeeIDdata + $cellend
$employeetype = $ObjUser.employeeType
$employeetypetitle = "Account Type: "
$employeetypedata = $employeetype
$TableBody = $TableBody + $defaultcolorCell + $employeetypetitle + $Cellmiddle2 + $employeetypedata + $cellend
#new Row
$TableBody = $TableBody + $TableRow
$location = $ObjUser.Office
$locationtitle = "Office: "
$locationdata = $location
$TableBody = $TableBody + $defaultcolorCell + $locationtitle + $Cellmiddle2 + $locationdata + $cellend
$email = $ObjUser.EmailAddress
$emailtitle = "Email: "
$emaildata = $email
$TableBody = $TableBody + $defaultcolorCell + $emailtitle + $Cellmiddle2 + $emaildata + $cellend
###########################################
###
### We need to pause and Get the multi DC stuff
###
##############################################
$LastLogintime = 0
$DefenderLastLogintime = 0
$DCLastFailedLogintime = 0
$BadLogins = 0
$accountchangedtime = 0
foreach($dc in $dcs)
{
$hostname = $dc.HostName
$LocalDCuser = Get-ADUser $UserSAM -Server $hostname | Get-ADObject -Properties *
if($LocalDCuser.'defender-lastLogon' -gt $DefenderLastLogintime)
{
$DefenderLastLogintime = $LocalDCuser.'defender-lastLogon'
}
if($LocalDCuser.LastLogon -gt $LastLogintime)
{
$LastLogintime = $LocalDCuser.LastLogon
}
if($LocalDCuser.BadPasswordTime -gt $DCLastFailedLogintime)
{
$DCLastFailedLogintime = $LocalDCuser.BadPasswordTime
}
if($LocalDCuser.whenChanged -gt $accountchangedtime)
{
$accountchangedtime = $LocalDCuser.whenChanged
}
if($LocalDCuser.BadLogonCount -gt $BadLogins)
{
[string]$BadLogins = $LocalDCuser.BadLogonCount
}
}
$LastSuccessfulLogin = [DateTime]::FromFileTime($LastLogintime)
$DefenderLastSuccessfulLogin = [DateTime]::FromFileTime($DefenderLastLogintime)
$lastfailedlogin = [DateTime]::FromFileTime($DCLastFailedLogintime)
$Acctchanged = $accountchangedtime
# Echo $username
# Echo "last logged on at: " $LastSuccessfulLogin
# Echo "last logged on with Defender token at: " $DefenderLastSuccessfulLogin
# Echo "last FAILED log on at: " $lastfailedlogin
# Echo "Recent Bad Logins: " $BadLogins
#
###################################################
# Oddball Account Properties
#new Line
$TableBody = $TableBody + $line
#new Row
$TableBody = $TableBody + $TableRow
$AcctCreated = $ObjUser.whenCreated
$AcctCreatedtitle = "Account Creation Date: "
$AcctCreateddata = $ObjUser.whenCreated
$TableBody = $TableBody + $defaultcolorCell + $AcctCreatedtitle + $Cellmiddle2 + $AcctCreateddata + $cellend
$Acctchangedtitle = "Account Last Changed: "
$Acctchangeddata = $Acctchanged
$TableBody = $TableBody + $defaultcolorCell + $Acctchangedtitle + $Cellmiddle2 + $Acctchangeddata + $cellend
###################################################
# Login times and Status
#new Line
$TableBody = $TableBody + $line
#Section header
$TableBody = $TableBody + "
LOGIN DATES |
"
#new Line
$TableBody = $TableBody + $line
#new Row
$TableBody = $TableBody + $TableRow
$LastSuccessfulLogintitle = "Last Successful Login: "
$LastSuccessfulLogindata = $LastSuccessfulLogin
$TableBody = $TableBody + $defaultcolorCell + $LastSuccessfulLogintitle + $Cellmiddle2 + $LastSuccessfulLogindata + $cellend
$DefenderLastSuccessfulLogintitle = "Last Defender Token Login: "
$DefenderLastSuccessfulLogindata = $DefenderLastSuccessfulLogin
$TableBody = $TableBody + $defaultcolorCell + $DefenderLastSuccessfulLogintitle + $Cellmiddle2 + $DefenderLastSuccessfulLogindata + $cellend
#new Row
$TableBody = $TableBody + $TableRow
$lastfailedlogintitle = "Last Failed Login: "
$lastfailedlogindata = $lastfailedlogin
$TableBody = $TableBody + $defaultcolorCell + $lastfailedlogintitle + $Cellmiddle2 + $lastfailedlogindata + $cellend
################################################################
## account status
#new Line
$TableBody = $TableBody + $line
#Section header
$TableBody = $TableBody + "
ACCOUNT STATUS |
"
#new Line
$TableBody = $TableBody + $line
#new Row
$TableBody = $TableBody + $TableRow
########## Account locked processing
$AcctLocked = $ObjUser.LockedOut
switch($AcctLocked)
{
True
{
$AccountLockedColor = "Red"
}
False
{
$AccountLockedColor = "LimeGreen"
}
}
$AcctLockedtitle = "Account Locked: "
$AcctLockeddata = $AcctLocked
$TableBody = $TableBody + $CellStart + $AccountLockedColor + $cellMiddle1 + $AcctLockedtitle + $Cellmiddle2 + $AcctLockeddata + $cellend
######### account control processing
$AccountControl = $ObjUser.userAccountControl
switch($AccountControl)
{
512
{
$accountDisabled = "False"
$accountDisabledColor = "LimeGreen"
$passwordexpires = "True"
$passwordexpirescolor = "LimeGreen"
}
514
{
$accountDisabled = "True"
$accountDisabledColor = "Red"
$passwordexpires = "True"
$passwordexpirescolor = "LimeGreen"
}
66048
{
$accountDisabled = "False"
$accountDisabledColor = "LimeGreen"
$passwordexpires = "false"
$passwordexpirescolor = "DarkOrange"
}
66050
{
$accountDisabled = "True"
$accountDisabledColor = "Red"
$passwordexpires = "false"
$passwordexpirescolor = "DarkOrange"
}
}
$accountDisabledtitle = "Account Disabled: "
$accountDisableddata = $accountDisabled
$TableBody = $TableBody + $CellStart + $accountDisabledColor + $cellMiddle1 + $accountDisabledtitle + $Cellmiddle2 + $accountDisableddata + $cellend
################################################################
## password status
#new Line
$TableBody = $TableBody + $line
#Section header
$TableBody = $TableBody + "
PASSWORD INFORMATION |
"
#new Line
$TableBody = $TableBody + $line
#new Row
$TableBody = $TableBody + $TableRow
$pwdlastChanged = $ObjUser.PasswordLastSet
$pwdlastChangedtitle = "Password Last Set: "
$pwdlastChangeddata = $pwdlastChanged
$TableBody = $TableBody + $defaultcolorCell + $pwdlastChangedtitle + $Cellmiddle2 + $pwdlastChangeddata + $cellend
########## password age calculation
$PwdAge = ($NOW - $pwdlastChanged).days
switch($PwdAge)
{
{$_ -ge 0 -and $_ -le 45}
{
$PwdAgeColor = "LimeGreen"
}
{$_ -ge 46 -and $_ -le 55}
{
$PwdAgeColor = "Yellow"
}
{$_ -ge 56 -and $_ -le 60}
{
$PwdAgeColor = "DarkOrange"
}
{$_ -ge 61}
{
$PwdAgeColor = "Red"
}
}
$PwdAgetitle = "Password Age: "
$PwdAgedata = $PwdAge
$TableBody = $TableBody + $CellStart + $PwdAgeColor + $cellMiddle1 + $PwdAgetitle + $Cellmiddle2 + $PwdAgedata + $cellend
#new Row
$TableBody = $TableBody + $TableRow
$passwordexpirestitle = "Password Expires Policy: "
$passwordexpiresdata = $passwordexpires
$TableBody = $TableBody + $CellStart + $passwordexpiresColor + $cellMiddle1 + $passwordexpirestitle + $Cellmiddle2 + $passwordexpiresdata + $cellend
$PwdExpired = $ObjUser.PasswordExpired
switch($PwdExpired)
{
False
{
$pwdExpiredColor = "LimeGreen"
}
True
{
$pwdExpiredColor = "Red"
}
}
$PwdExpiredtitle = "Password Expired?: "
$PwdExpireddata = $PwdExpired
$TableBody = $TableBody + $CellStart + $PwdExpiredColor + $cellMiddle1 + $PwdExpiredtitle + $Cellmiddle2 + $PwdExpireddata + $cellend
#new Row
$TableBody = $TableBody + $TableRow
$UserchangePwd = $ObjUser.CannotChangePassword
switch($UserchangePwd)
{
False
{
$UserChangepwdColor = "LimeGreen"
$UserchangePwdStatus = "Yes"
}
True
{
$UserChangepwdColor = "Yellow"
$UserchangePwdStatus = "No"
}
}
$UserchangePwdtitle = "User can change password?: "
$UserchangePwddata = $UserchangePwdStatus
$TableBody = $TableBody + $CellStart + $UserchangePwdColor + $cellMiddle1 + $UserchangePwdtitle + $Cellmiddle2 + $UserchangePwddata + $cellend
# Domain Password Policy stuff
$ADDomainPasswordPolicy = Get-ADDefaultDomainPasswordPolicy
#new Row
$TableBody = $TableBody + $TableRow
[string]$maxattempts = $ADDomainPasswordPolicy.LockoutThreshold
[string]$Remainingattempts = $maxattempts - $BadLogins
$maxattemptstitle = "Max/Remaining Password Attempts: "
[string]$maxattemptsdata = $maxattempts + " / " + $Remainingattempts
$TableBody = $TableBody + $defaultcolorCell + $maxattemptstitle + $Cellmiddle2 + $maxattemptsdata + $cellend
########## calculate account unlock policy
$LockoutDuration = $ADDomainPasswordPolicy.LockoutDuration
if ($LockoutDuration -le 0)
{
$Autounlock = "Manual Unlock Only"
}
if ($LockoutDuration -gt 0)
{
$Autounlock = "[Days:Hours:Minutes] " + $LockoutDuration
}
$Autounlocktitle = "Auto Unlock Duration: "
$Autounlockdata = $Autounlock
$TableBody = $TableBody + $defaultcolorCell + $Autounlocktitle + $Cellmiddle2 + $Autounlockdata + $cellend
#new Row
$TableBody = $TableBody + $TableRow
$MinPwdlength = $ADDomainPasswordPolicy.MinPasswordLength
$MinPwdlengthtitle = "Minimum Password Length: "
$MinPwdlengthdata = $MinPwdlength
$TableBody = $TableBody + $defaultcolorCell + $MinPwdlengthtitle + $Cellmiddle2 + $MinPwdlengthdata + $cellend
$MinPwdHistory = $ADDomainPasswordPolicy.PasswordHistoryCount
$MinPwdHistorytitle = "Minimum Password History: "
$MinPwdHistorydata = $MinPwdHistory
$TableBody = $TableBody + $defaultcolorCell + $MinPwdHistorytitle + $Cellmiddle2 + $MinPwdHistorydata + $cellend
################################################################
## Profile Information
#new Line
$TableBody = $TableBody + $line
#Section header
$TableBody = $TableBody + "
PROFILE INFORMATION |
"
#new Line
$TableBody = $TableBody + $line
#new Row
$TableBody = $TableBody + $TableRow
$Script = $ObjUser.ScriptPath
$Scripttitle = "Login Script: "
$Scriptdata = $Script
$TableBody = $TableBody + $defaultcolorCell + $Scripttitle + $Cellmiddle2 + $Scriptdata + $cellend
#new Row
$TableBody = $TableBody + $TableRow
$homeLocation = $ObjUser.l
$HomeDirectory = "\\microsoft.com\user\" + $homeLocation + "\" + $UserSAM + "\"
$HomeDirectorytitle = "Home Drive: "
$HomeDirectorydata = $HomeDirectory
$TableBody = $TableBody + $defaultcolorCell + $HomeDirectorytitle + $Cellmiddle2 + $HomeDirectorydata + $cellend
#new Row
$TableBody = $TableBody + $TableRow
$CitrixProfile = "\\micosoft.com\citrix\Profiles\TS\" + $UserSAM +"
" + "\\microsoft.com\citrix\Profiles\TS-x64\" + $UserSAM
$CitrixProfiletitle = "Citrix Profiles: "
$CitrixProfiledata = $CitrixProfile
$TableBody = $TableBody + $defaultcolorCell + $CitrixProfiletitle + $Cellmiddle2 + $CitrixProfiledata + $cellend
################################################################
## Exchange information
#new Line
$TableBody = $TableBody + $line
#Section header
$TableBody = $TableBody + "
EXCHANGE INFORMATION |
"
#new Line
$TableBody = $TableBody + $line
#new Row
$TableBody = $TableBody + $TableRow
$ExchangeAccount = $ObjUser.msExchWhenMailboxCreated
$ExchangeAccounttitle = "Mailbox Created: "
$ExchangeAccountdata = $ExchangeAccount
$TableBody = $TableBody + $defaultcolorCell + $ExchangeAccounttitle + $Cellmiddle2 + $ExchangeAccountdata + $cellend
#new Row
$TableBody = $TableBody + $TableRow
$proxyaddresses = $ObjUser.proxyAddresses
Foreach ($mailitem in $proxyaddresses)
{
$mailitemlist = $mailitemlist + "
" + $mailitem
}
$proxyaddressestitle = "Messaging Addresses: "
$proxyaddressesdata = $mailitemlist
$TableBody = $TableBody + $defaultcolorCell + $proxyaddressestitle + $Cellmiddle2 + $proxyaddressesdata + $cellend
#new Row
$TableBody = $TableBody + $TableRow
$AssignedDelegates = $ObjUser.publicDelegatesBL
Foreach ($mailbox in $AssignedDelegates)
{
$objmailbox = Get-ADUser -Identity $mailbox
$mailboxname = $objmailbox.name
$Publicmailbox = $publicmailbox + "
" + $mailboxname
}
$AssignedDelegatestitle = "Assigned Delegates: "
$AssignedDelegatesdata = $Publicmailbox
$TableBody = $TableBody + $defaultcolorCell + $AssignedDelegatestitle + $Cellmiddle2 + $AssignedDelegatesdata + $cellend
$mailboxmanager = $ObjUser.msExchDelegateListBL
Foreach ($mailbox in $mailboxmanager)
{
$objmailbox = Get-ADUser -Identity $mailbox
$mailboxname = $objmailbox.name
$mailboxlist = $mailboxlist + "
" + $mailboxname
}
$mailboxmanagertitle = "Delegate Access: "
$mailboxmanagerdata = $mailboxlist
$TableBody = $TableBody + $defaultcolorCell + $mailboxmanagertitle + $Cellmiddle2 + $mailboxmanagerdata + $cellend
############################
##
## Managers Data to return
##
############################
################################################################
## Manager information
#new Line
$TableBody = $TableBody + $line
#Section header
$TableBody = $TableBody + "
LINE MANAGER |
"
#new Line
$TableBody = $TableBody + $line
#new Row
$TableBody = $TableBody + $TableRow
$manager = $ObjUserManager.Name
$managertitle = "Manager Name: "
$managerdata = $manager
$TableBody = $TableBody + $defaultcolorCell + $managertitle + $Cellmiddle2 + $managerdata + $cellend
#new Row
$TableBody = $TableBody + $TableRow
$managertitle = $ObjUserManager.title
$managertitletitle = "Manager Title: "
$managertitledata = $managertitle
$TableBody = $TableBody + $defaultcolorCell + $managertitletitle + $Cellmiddle2 + $managertitledata + $cellend
$managerphone = $ObjUserManager.OfficePhone
$managerphonetitle = "Manager Office Phone: "
$managerphonedata = $managerphone
$TableBody = $TableBody + $defaultcolorCell + $managerphonetitle + $Cellmiddle2 + $managerphonedata + $cellend
###################################
##
## Message
##
#############################################################################
$Message = $Message + $tableHeader + $Tablebody + $tableend
This gives us pretty output we can send to users. I hope it helps.
Sending email is a common task.. here is a simple but functional …Function 🙂
function sendMail($BODYemail,$SUBemail,$TOemail) {
Write-Host "Sending Email"
#SMTP server name
$smtpServer = "smtpserver.MYDOMAIN.COM"
#Creating a Mail object
$msg = new-object Net.Mail.MailMessage
#Creating SMTP server object
$smtp = new-object Net.Mail.SmtpClient($smtpServer)
#Email structure
$msg.From = "FROMEMAIL@MYDOMAIN.COM"
$msg.ReplyTo = "REPLYTOEMAIL@MYDOMAIN.COM"
$msg.To.Add("$TOemail")
$msg.subject = "$SUBemail"
$msg.body = "$BODYemail"
$msg.IsBodyHTML = $true
#Sending email
$smtp.Send($msg)
}
Send some info
# To the User
$TOemail = "$OBJ_ContactPointEmail"
$BODYemail = $MSG_Header + $MSG_USERBODY + $MSG_Foot
$SUBemail = "Follow-up information on your request [$INVAR_SR]"
sendMail $BODYemail $SUBemail $TOemail
If ($INT_CountSAG -ne 0 )
{
$TOemail = "MYEMAIL@MYDOMAIN.COM"
$BODYemail = $SAGMessage
$SUBemail = "SAG Follow up"
sendMail $BODYemail $SUBemail $TOemail
}
If ($INT_CountErrors -ne 0 )
{
$TOemail = "MYEMAIL@MYDOMAIN.COM"
$BODYemail = $MSG_ERRORBODY + $MSG_SAGADMIN
$SUBemail = "Add users to groups errors"
sendMail $BODYemail $SUBemail $TOemail
}

When I first heard about PowerShell (an eternity ago), I basically ignored it. I thought – “My Batch files and VBScripts work just fine.”
However – as more and more Microsoft tools began to transition to utilizing PowerShell I took it upon myself to learn.
Now.. I can’t imaging going back to simple VBScript (.. I admit I still have some VB I still use.. because those scripts still work!)
So how does one get started learning PowerShell?
1. Pick a project: You need a project in order to focus your mind. If you just try to jump out and read commands – all that will do is familiarize you, and that’s essential tool, but it won’t get it cemented into your brain. So think of something simple you want to do. For our sample – lets do something simple:
- Get all the people in an active directory group.
2. Type it up: In your mind, break down the steps of what you want to do in as many steps as you can think of – think like a computer, what does it need to do to get the info you want. For our example:
- define a group name to a variable
- query ad for the group
- look up the members of the group
- display the members
3. Documented Code: One of the nice thing about doing this is that it helps you formulate your thoughts on what needs to happen in small workable chunks. Another is that you can use your comments as the beginning of comments for your code. It’s always important to comment your code, because when others review it – it will help them follow along…. including when that other is yourself 6 months later trying to revise your own code! In powershell we use the # sign to document a comment
- # define a group name to a variable
- # query ad for the group
- # look up the members of the group
- # display the members
4. Replace ideas with Code: The next step is to replace the ideas – with actual powershell. Don’t hesitate to use your favorite search engine and look up how to do each component.
# define a group name to a variable
$MYGROUPNAME = "Test-Group"
# query ad for the group
$MYGROUPOBJECT = get-adgroup -Identity $MYGROUPNAME -Properties *
# look up the members of the group
$MYGROUPMEMBERS = $MYGROUPOBJECT.members
# display the members
echo $MYGROUPMEMBERS
This is a simple script (and it could be a lot simpler – one line in fact), but as you learn – the principles of how to learn stay the same. Break it down into smaller components and take it one section at a time until you are familiar with coding in PowerShell!
… so like any homeowner I’ve had to fix a toilet or two in my time. When a task comes up at work – I am the guy who is always saying “stop and do it right the first time”… so of course I am also the guy who doesn’t take his own advice on household chores.
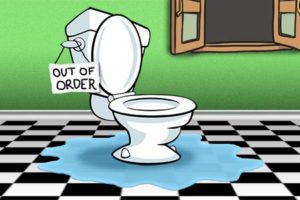
So back to my toilet. The tank was constantly running. It’s a 15 year old toilet so I knew right away that the parts in the tank with the “5 year warranty” probably needed replacing… Call me “Powers of Deduction Man!” – my superpower is knowing the obvious.
So I run off to my local Home Depot and get a kit… do i get complete kit? nah. I grab the kit with just the most common pieces.
An hour later i’ve replaced the parts, and while things are better – I have a slow leak from the top tank into the bowl (not the floor thankfully). Which I attempt to patch. Six months later… my leak is back. And it quickly gets … worse.
So back to Home depot for the … other half of the kit. So kids – the lesson here..
“Stop and do it right the first time”